Bu yazımızda C# form örneği olarak hesap makinesi yapıyor olacağız. Bundan yıllar önce lisede yazılım dersinde C++ dilinde hesap makinesi tasarlamıştım. Güzel günlerdi. Bu gün yapacağımız hesap makinesi hesap geçmişini tutan orta profesyonel bir yazılım olacak.
1. C# Hesap Makinesi Form Tasarımı
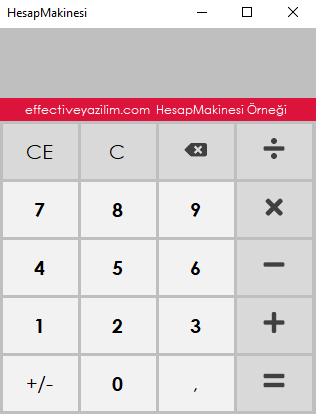
C# form örnekleri – Hesap makinesi tasarımını yaparken ikonlu buton kullandım. ikon buton kullanımını anlattığımız yazımıza BURADAN ulaşabilirsiniz.
Form üzerinde kullandığımız 2 adet textbox var ve bunları sadece okunabilir olarak işaretledik. Kullanılan bütün butonların isimlerini aldıkları göreve göre değiştirdik.
2. C# Hesap makinesi kodlama
Form tasarımınızı bitirdiyseniz kodlamaya geçebiliriz. İlk olarak değişkenlerimizi ekliyoruz.
double? gecmis = 0, sonuc, sayi;
string sonsayi,islem;
bool hareket;
Rakamları barındıran butonların kodlarını bir metot içerisinde kodlayacağız.
void Sayilar(string s)
{
hareket = true;
if (sonsayi == "0")
sonsayi = s;
else
sonsayi += s;
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble(sonsayi);
}
Şimdide bütün rakamların click eventine aşağıdaki kodu yazalım. Burada dikkat etmemiz gereken şey hangi rakamın butonuna basıyorsak o rakamı metoda göndermeliyiz. Örneğin 6 butonuna bastıysak aşağıdaki gibi olacaktır. Not: Sıfır butonuna da bu kodu yazıyoruz.
Sayilar("6");
Not: C# ve veya operatörlerini anlattığımız yazımıza BURADAN ulaşabilirsiniz. Yazımızın sonunda tüm form kodları mevcuttur.
Değeri artı değer veya eksi değere döndürmek için +/- butonuna bu kodu yazıyoruz.
if (txSonuc.Text == "0" || txSonuc.Text == "")
txSonuc.Text = "0";
else
{
sonsayi = (Convert.ToDouble(txSonuc.Text) * -1).ToString();
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble(sonsayi);
}
Not: C# sayıları negatife veya pozitife dönüştürme yazımıza BURADAN ulaşabilirsiniz.
Eğer değerimize ondalık sayı eklemek istersek Virgül olan buton içerisine aşağıdaki kodu yazıyoruz ve böylece girdiğimiz değerin sonuna virgül eklemiş oluyoruz. Burada Contains – Değer içerisinde arama yapma kodunu kullandık. Yöntemi buradan inceleyebilirsiniz.
bool _bool = txSonuc.Text.Contains(",");
if (txSonuc.Text == "0" || txSonuc.Text == "")
txSonuc.Text = "0";
else
{
if (_bool != true)
{
sonsayi += ",";
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble(sonsayi);
}
}
Şimdide değerden tek tek silme tuşumuzu ayarlayalım. Görüldüğü gibi String değerimizde son karakteri silmek için SubString komutu kullandık.
if (txGecmis.Text != "")
islem = txGecmis.Text.Substring(txGecmis.Text.Length - 2, 2);
if (txSonuc.Text == "0")
txSonuc.Text = "0";
else
{
if(islem==" =")
{
txGecmis.Text = "";
gecmis = 0; sonuc = null; sayi = null;
sonsayi = "";
islem = "";
hareket = false;
}
else
{
if (txSonuc.Text.Length > 1)
sonsayi = txSonuc.Text.Substring(0, txSonuc.TextLength - 1);
else sonsayi = "0";
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble(sonsayi);
}
}
CE butonumuzun click eventinin kodlarını yazalım.
txSonuc.Text = "0";
C butonumuzun click eventinin kodlarını yazalım.
txSonuc.Text = "0";
txGecmis.Text = "";
gecmis =0; sonuc = null; sayi = null;
sonsayi = "";
islem = "";
hareket = false;
Önemli : Hesap makinesi işlemlerinin kodlaması
Geldik Toplama, Çıkarma, Çarpma ve Bölme işlemlerini yapmaya. Ve burada da metot yazıp butonlardan bu metodu çağırıyor olacağız.
void islemler(string i)
{
if (txGecmis.Text != "")
islem = txGecmis.Text.Substring(txGecmis.Text.Length - 2, 2);
else
islem = i;
if (gecmis != 0)
{
if (hareket == true)
{
if (islem == " +")
sonuc = gecmis + sayi;
else if (islem == " -")
sonuc = gecmis - sayi;
else if (islem == " x")
sonuc = gecmis * sayi;
else if (islem == " ÷")
sonuc = gecmis / sayi;
hareket = false;
}
}
else
{
if (sayi == null)
sayi = 0;
sonuc = sayi;
}
if (islem == " =")
islem = i;
sonsayi = "0";
gecmis = sonuc;
txGecmis.Text = sonuc + i;
txSonuc.Text = sonuc.ToString();
}
Görüldüğü üzere yapılan işlemi islem adında bir değişkene atıyoruz. Yapılacak olan işlemi de bu değişken sayesinde belirliyoruz. Bu yüzden işlem göndereceğimiz butonlarda bu metodu çağırırken işlemin ne olduğunu belirtmemiz gerekiyor. bir örnek vermek gerekirse;
islemler(" +");
ve Son olarak eşittir butonuna tıklandığında çalışacak olan kodu yazalım.
if (islem == " +")
sonuc = gecmis + sayi;
else if (islem == " -")
sonuc = gecmis - sayi;
else if
(islem == " x")
sonuc = gecmis * sayi;
else if
(islem == " ÷")
sonuc = gecmis / sayi;
else
{
sonuc = sayi;
gecmis = null;
}
sonsayi = "0";
txGecmis.Text = gecmis + islem + sayi + " =";
gecmis = sonuc;
txSonuc.Text = sonuc.ToString();
Sonuç

Bildiğinizi var sayarak her satırı açıklamadım. Fakat yorumlarda belirtirseniz açıklamalar ekleyebilirim. Dilerseniz projeyi de paylaşabilirim. Geliştirme isterseniz klavye tuşlarını atama yapabiliriz. Şimdilik bu kadar olsun. İyi çalışmalar.
C# hesap makinesi kodun tamamı
using System;
using System.Windows.Forms;
namespace uygulama_anlatım_hesap_makinesi
{
public partial class HesapMakinesi : Form
{
public
HesapMakinesi()
{
InitializeComponent();
}
double? gecmis = 0, sonuc, sayi;
string sonsayi,islem;
bool hareket;
void Sayilar(string s)
{
hareket = true;
if
(sonsayi == "0")
sonsayi = s;
else
sonsayi += s;
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble
(sonsayi);
}
void
islemler(string i)
{
if
(txGecmis.Text != "")
islem = txGecmis.Text.Substring(txGecmis.Text.Length - 2, 2);
else
islem = i;
if
(gecmis != 0)
{
if
(hareket == true)
{
if
(islem == " +")
sonuc = gecmis + sayi;
else if
(islem == " -")
sonuc = gecmis - sayi;
else if
(islem == " x")
sonuc = gecmis * sayi;
else if
(islem == " ÷")
sonuc = gecmis / sayi;
hareket = false;
}
}
else
{
if
(sayi == null)
sayi = 0;
sonuc = sayi;
}
if
(islem == " =")
islem = i;
sonsayi = "0"
;
gecmis = sonuc;
txGecmis.Text = sonuc + i;
txSonuc.Text = sonuc.ToString
();
}
private void
btnArtiEksi_Click(object
sender, EventArgs
e)
{
if
(txSonuc.Text == "0" || txSonuc.Text == ""
)
txSonuc.Text = "0"
;
else
{
sonsayi = (Convert.ToDouble(txSonuc.Text) * -1).ToString
();
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble
(sonsayi);
}
}
private void
btnVirgul_Click(object
sender, EventArgs
e)
{
bool _bool = txSonuc.Text.Contains(",");
if
(txSonuc.Text == "0" || txSonuc.Text == "")
txSonuc.Text = "0";
else
{
if
(_bool != true)
{
sonsayi += ",";
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble
(sonsayi);
}
}
}
private void
btnSilTekRakam_Click(object
sender, EventArgs
e)
{
if
(txGecmis.Text != "")
islem = txGecmis.Text.Substring(txGecmis.Text.Length - 2, 2);
if
(txSonuc.Text == "0"
)
txSonuc.Text = "0";
else
{
if
(islem==" =")
{
txGecmis.Text = "";
gecmis = 0; sonuc = null; sayi = null;
sonsayi = "";
islem = "";
hareket = false;
}
else
{
if
(txSonuc.Text.Length > 1)
sonsayi = txSonuc.Text.Substring(0, txSonuc.TextLength - 1);
else
sonsayi = "0"
;
txSonuc.Text = sonsayi;
sayi = Convert.ToDouble(sonsayi);
}
}
}
private void
btnCE_Click(object
sender, EventArgs
e)
{
txSonuc.Text = "0";
}
private void
btnC_Click(object
sender, EventArgs
e)
{
txSonuc.Text = "0";
txGecmis.Text = "";
gecmis =0; sonuc = null
; sayi = null
;
sonsayi = "";
islem = "";
hareket = false;
}
private void
btnEsittir_Click(object
sender, EventArgs
e)
{
if (islem == " +")
sonuc = gecmis + sayi;
else if
(islem == " -")
sonuc = gecmis - sayi;
else if
(islem == " x")
sonuc = gecmis * sayi;
else if (islem == " ÷")
sonuc = gecmis / sayi;
else
{
sonuc = sayi;
gecmis = null;
}
sonsayi = "0";
txGecmis.Text = gecmis + islem + sayi + " =";
gecmis = sonuc;
txSonuc.Text = sonuc.ToString();
}
private void
btnTopla_Click(object
sender, EventArgs
e)
{
islemler
(" +");
}
private void
btnCikar_Click(object
sender, EventArgs
e)
{
islemler
(" -");
}
private void
btnCarp_Click(object
sender, EventArgs
e)
{
islemler
(" x");
}
private void
btnBol_Click(object
sender, EventArgs
e)
{
islemler(" ÷");
}
private void
btn1_Click(object
sender, EventArgs
e)
{
Sayilar
("1");
}
private void
btn2_Click(object
sender, EventArgs
e)
{
Sayilar
("2");
}
private void
btn3_Click(object
sender, EventArgs
e)
{
Sayilar
("3");
}
private void
btn4_Click(object
sender, EventArgs
e)
{
Sayilar
("4");
}
private void
btn5_Click(object
sender, EventArgs
e)
{
Sayilar
("5");
}
private void
btn6_Click(object
sender, EventArgs
e)
{
Sayilar
("6");
}
private void
btn7_Click(object
sender, EventArgs
e)
{
Sayilar
("7");
}
private void
btn8_Click(object
sender, EventArgs
e)
{
Sayilar
("8");
}
private void
btn9_Click(object
sender, EventArgs
e)
{
Sayilar
("9");
}
private void
btn0_Click(object sender, EventArgs e)
{
Sayilar("0");
}
}
}
Bir yanıt yazın