Bu yazımızda c# ile sql işlemlerini örneklerle anlatıyor olacağız.
Öğrenmenin en iyi yolu olan örneklemeyle başlayalım. Diyelim ki öğrenci kayıt sistemi yapıyoruz ve sql ile çalışmayı planlıyoruz.
Öncelikle Mssql de okul adında bir veri tabanı oluşturdum. Bu veritabanının içine ogrenci diye bir tablo oluşturdum.

Gelelim C# üzerinde formumuzu tasarlamaya;

Görüldüğü üzere form üzerinde 4 textbox 3 button ve birde datagridview bulunmakta. artık kodlara geçebiliriz…
İlk olarak usinglerimizi ekleyelim
using System.Data; using System.Data.SqlClient;
Sql bağlantı cümlemizi sql adapteri datatable yi ve sql commandı atayalım
Sql Bağlantı cümlelerini detaylı incelemek isterseniz blog yazımıza buradan ulaşabilirsiniz.
//Windows Authentication ile localde kurulu servere bağlantı yapıyoruz. SqlConnection con = new SqlConnection("Data Source=.; Initial Catalog=okul; Integrated ecurity=True; Persist Security Info=False"); SqlDataAdapter da; SqlCommand cmd; DataTable dt = new DataTable();
Öğrencileri datagridview de listelemek için bir void metodu yazalım.
C# Datagridview sql üzerinden veri çekme işlemini anlattığımız yazımızı buradan inceleyebilirsiniz.
void Listele() { // listele methodu oluşturduk ve sql ogrenci tablosundaki her veriyi datagridviewe aktardık da = new SqlDataAdapter("Select * From ogrenci", con); con.Open(); da.Fill(dt); dataGridView1.DataSource = dt; con.Close(); }
şimdi sırasıyla butonların kodlarını paylaşacağım.
private void btnEkle_Click(object sender, EventArgs e) { //textboxların boşmu dolumu olduğunu kontrol ettik eğer boşlarsa massagebox ozelliğini ile kullanıcıya uyarı gönderdik - eğer textboxlar doluysa veritabanına ekleme işlemini gerçekleştirdik if (string.IsNullOrEmpty(txNo.Text) && string.IsNullOrEmpty(txAd.Text) && string.IsNullOrEmpty(txSoyad.Text) && string.IsNullOrEmpty(txTel.Text)) { MessageBox.Show("Eksik yerler var!"); } else { cmd = new SqlCommand("insert into ogrenci(no,ad,soyad,telefon) values ('" + txNo.Text + "','" + txAd.Text + "','" + txSoyad.Text + "','" + txTel.Text + "')", con); con.Open(); cmd.ExecuteNonQuery(); con.Close(); } // oluşturduğumuz listele methodunu çağırdık Listele(); }
private void btnGuncelle_Click(object sender, EventArgs e) { // textboxların boşmu dolumu olduğunu kontrol ettik eğer textboxlar doluysa veritabanına güncelle işlemini gerçekleştirdik if (string.IsNullOrEmpty(txNo.Text) && string.IsNullOrEmpty(txAd.Text) && string.IsNullOrEmpty(txSoyad.Text) && string.IsNullOrEmpty(txTel.Text)) { MessageBox.Show("Eksik yerler var!"); } else { cmd = new SqlCommand("update ogrenci set ad='" + txAd.Text + "',soyad='" + txSoyad.Text + "',telefon='" + txTel.Text + "' where no='" + txNo.Text + "'", con); con.Open(); cmd.ExecuteNonQuery(); con.Close(); } Listele(); }
private void btnSil_Click(object sender, EventArgs e) { // öğrenci no boşmu dolumu olduğunu kontrol ettik doluysa tablodan sil işlemini gerçekleştirdik if (string.IsNullOrEmpty(txNo.Text) && string.IsNullOrEmpty(txAd.Text) && string.IsNullOrEmpty(txSoyad.Text) && string.IsNullOrEmpty(txTel.Text)) { MessageBox.Show("Eksik yerler var!"); } else { cmd = new SqlCommand("delete from ogrenci where no='" + txNo.Text + "'", con); con.Open(); cmd.ExecuteNonQuery(); con.Close(); } Listele(); }
Burada if kontrolunu IsNullOrEmpty ile kullanmış bulunmaktayım. Bu işlemi kısaca anlatmak gerekirse textbox içerisinde bir değer varmı sorusunu sormaktayız.
if else kullanımını anlattığımız yazımıza buradan ulaşabilirsiniz.
isnullorempty kullanımını anlattımız yazımıza buradan ulaşabilirsiniz.
Kodun tamanı bu şekilde olmalı
using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Data; using System.Data.SqlClient; namespace ogrenci_kayit_sistemi { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { Listele(); } SqlConnection con = new SqlConnection("Data Source=.; Initial Catalog=okul; Integrated ecurity=True; Persist Security Info=False"); SqlDataAdapter da; SqlCommand cmd; DataTable dt = new DataTable(); void Listele() { da = new SqlDataAdapter("Select * From ogrenci", con); con.Open(); da.Fill(dt); dataGridView1.DataSource = dt; con.Close(); } private void btnEkle_Click(object sender, EventArgs e) { if(string.IsNullOrEmpty(txNo.Text)&& string.IsNullOrEmpty(txAd.Text)&& string.IsNullOrEmpty(txSoyad.Text)&& string.IsNullOrEmpty(txTel.Text)) { MessageBox.Show("Eksik yerler var!"); } else { cmd = new SqlCommand("insert into ogrenci(no,ad,soyad,telefon) values ('" + txNo.Text + "','" + txAd.Text + "','" + txSoyad.Text + "','" + txTel.Text + "')", con); con.Open(); cmd.ExecuteNonQuery(); con.Close(); } Listele(); } private void btnGuncelle_Click(object sender, EventArgs e) { if(string.IsNullOrEmpty(txNo.Text)&& string.IsNullOrEmpty(txAd.Text)&& string.IsNullOrEmpty(txSoyad.Text)&& string.IsNullOrEmpty(txTel.Text)) { MessageBox.Show("Eksik yerler var!"); } else { cmd = new SqlCommand("update ogrenci set ad='" + txAd.Text + "',soyad='" + txSoyad.Text + "',telefon='" + txTel.Text + "' where no='" + txNo.Text + "'", con); con.Open(); cmd.ExecuteNonQuery(); con.Close(); } Listele(); } private void btnSil_Click(object sender, EventArgs e) { if(string.IsNullOrEmpty(txNo.Text)&& string.IsNullOrEmpty(txAd.Text)&& string.IsNullOrEmpty(txSoyad.Text)&& string.IsNullOrEmpty(txTel.Text)) { MessageBox.Show("Eksik yerler var!"); } else { cmd = new SqlCommand("delete from ogrenci where no='" + txNo.Text + "'", con); con.Open(); cmd.ExecuteNonQuery(); con.Close(); } Listele(); } } }
Ve sonuç ;
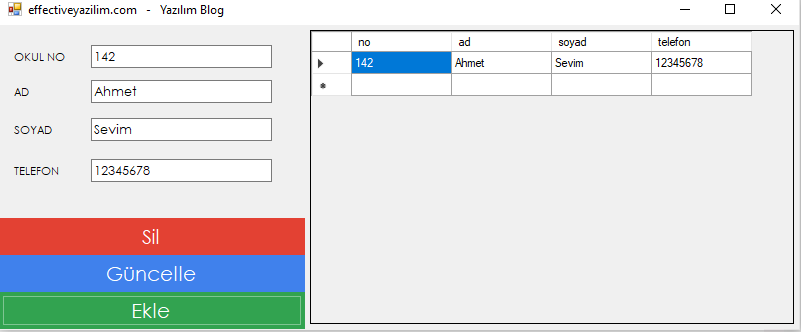
Başka yazılarda görüşmek dileğiyle iyi çalışmalar..
Bir yanıt yazın